CMSC 435/634: Computer Graphics
Lab 6: Ray tracing
Due December 7, 2000
Note: There is a bug in the Windows version of the edge library
EdgeDrawScanline function Below is the correct glDrawPixels
command
glDrawPixels( NumPixels, 1, GL_RGBA, GL_FLOAT, Scanline );
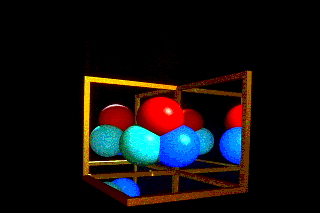
Purpose:
To gain experience in 3D hiddens surface removal and rendering using
a simple raytracer.
Assignment
Implement a program that will allow defining spheres in a world space and
viewing any part of it in screen space.
Implement the following commands.
-
observer_position: posX posY posZ or observer: posX
posY posZ
-
coi: coiX coiY coiZ
-
head_tilt: tilt
hither_yon: hither yon
-
view_angle: angle or angle: angle
-
Position the observer in world space and set the viewing parameters: observer
position, center-of-interest, head tilt (angle), hither and yon clipping
distances, and horizontal half-angle-of-view.
-
viewport: vxl vxr vyb vyt
-
Clip and display the visible lines in a viewport on the screen defined
by the four parameters. The four values are, in order, x-left, x-right,
y-bottom, y-top limits of the world window and screen viewport.
-
device: keyword
-
input either the word ``screen'' (for drawing to the screen or a filename
to write the image to a file.
- PPM File Format:
-
-     P3
-
-     xsize ysize
-
-     255
-
-     r g b r g b r g b r g b .... r g b \n
-
-     r g b r g b r g b r g b .... r g b \n
-
-     r g b r g b r g b r g b .... r g b \n
-
-     r g b r g b r g b r g b .... r g b \n
-
- where r g b are int's between 0 and 255
-
shadows: 1
-
calculate shadows
-
shadows: 0
-
don't calculate shadows
-
reflections: 1
-
calculate reflections
-
refraction: 1
-
calculate refracted rays
-
light_position: x y z
-
set light source position.
1 or more commands of the form:
-
sphere: red green blue cx cy cz radius
-
Position a sphere in world space of radius radius at location
(cx,cy,cy)
with color (red,green,blue)
-
illumination_parameters: ka kd ks exp
-
set illumination parameters for ambient, diffuse, specular, and specular
exponent. Note: this is per sphere.
-
texture_function: function_name (optional command)
-
a function to texture map some
shading parameter of the object.
-
-
end or <end of file>
-
Terminates the program
-
#
-
Ignore the rest of the line; this is a comment line
Notes
-
View calculations
Perform your ray-casting in world space. Initially, you may assume
the observer is at the origin looking down the negative Z-axis as in
the following diagram:
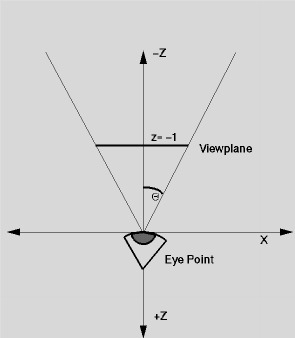
- To handle the other cases, translate and rotate the objects in
the scene based on the observer's position and center of interest
(translate the observer to the origin and rotate the center of
interest to be along the -Z axis). Then, rotate about Z to handle head
tilt.
-
To calculate the field of view,
assume that your rays go from
-
X: -tan(theta) to tan(theta)
-
Y: ratio*-tan(theta) to ratio*tan(theta), where
-
ratio = (Vyt - Vyb)/(Vxr - Vxl)
-
When calculating shadows, be careful not to intersect the sphere
you're on when going away from it towards a light source, i.e., intersecting
it at the point of origin before you ever leave. You can test to make sure
the center point of a sphere you're about to intersect is in front of you;
You can just skip over the test for the ith sphere, if that's the one you're
on; Or you can add some epsilon value that you have to be beyond, before
you count it as a real intersection (this can create problems with two
touching spheres if you're not careful).
Also, notice that the shadow intersection has to be with the
semi-infiinte ray, not the infinite line. That is, the
intersection has to be out in front of the point of origin, not behind
it.
-
You can limit the world data structure to a maximum of 50 objects.
-
You need to create a procedural texture function for simulating wood
objects. If you use any algorithms that you don't create, fully document
where they came from. You must also supply an input file for this
procedure.
-
Defaults: ka = 0.2; kd = .8; ks = 1.0; exp =50; no shadows; no
reflections, no refraction
-
The lab should read the commands from standard input or have a button
to read an input file.
Extra Credit
-
25% for implementing polygon intersections and adding the ability to read
in .det files. REQUIRED FOR CMSC 634 STUDENTS
-
15% each for a good attempt at water, fireball, or cloud
Suggestions
Implement features in the following order:
-
Get visibility working using just write a white pixel whenever an
object is hit by the pixel ray
-
add intensity calculation and then color.
-
add shadows.
-
add reflections
David S. Ebert